Triangle fan opengl draw
Table of Contents
Table of Contents
If you’re interested in programming and want to learn how to create graphics using c++, then you’ve come to the right place. One of the most fundamental shapes in geometry is a triangle, and it is a great way to start your journey. Let’s explore it together!
Introduction
As a beginner, diving into graphics programming using c++ can be challenging. Initial thoughts can be clouded by codes and techniques. Though several libraries like OpenGL can be used to facilitate graphics programming, learning how to code it manually is very important. Drawing a triangle is an essential and foundational practice for graphics programming in c++. The reason behind it is that every complex shape is constructed by combining a series of triangles.
How to Draw a Triangle in C++
When it comes to c++ programming, drawing a triangle is quite simple because it only involves a few lines of code.
To begin, you need to define your vertices. A vertex is a point or coordinate in space. In this case, we only need three vertices to create a triangle. The vertices are defined as follows:
float vertices\[\] = -0.5f, -0.5f, 0.0f, 0.5f, -0.5f, 0.0f, 0.0f, 0.5f 0.0f ;
The above code defines a set of three vertices that form a triangle.
Now we need to render those vertices on the screen. To do this, we need a vertex array object (VAO) and a vertex buffer object (VBO) to store our vertices. Here are the necessary lines of code:
unsigned int VAO, VBO; glGenVertexArrays(1, &VAO); glGenBuffers(1, &VBO); glBindVertexArray(VAO); glBindBuffer(GL\_ARRAY\_BUFFER, VBO); glBufferData(GL\_ARRAY\_BUFFER, sizeof(vertices), vertices, GL\_STATIC\_DRAW); glVertexAttribPointer(0, 3, GL\_FLOAT, GL\_FALSE, 3 \* sizeof(float), (void\*)0); glEnableVertexAttribArray(0); glBindBuffer(GL\_ARRAY\_BUFFER, 0); glBindVertexArray(0);
The above block of code generates a VAO and VBO, binds them, buffers data, specifies vertex attribute pointers, enables them, and binds VBO and VAO to 0.
Finally, to actually draw the triangle, you need the following lines of code:
while (!glfwWindowShouldClose(window)) glClear(GL\_COLOR\_BUFFER\_BIT); glBindVertexArray(VAO); glDrawArrays(GL\_TRIANGLES, 0, 3); glfwSwapBuffers(window); glfwPollEvents();
The above code executes a game loop that clears and redraws the triangle at each frame.
Summary on How to Draw a Triangle in C++
To summarize the above, we need to accomplish the following steps:
- Define the vertices
- Generate a VAO and VBO, bind them, buffer the data, and specify vertex attribute pointers
- Draw the triangle in a loop.
Why Draw a Triangle Using Multiple Triangles?
OpenGL renders triangles in 3D, however, we outline a 2D triangle. When we draw a triangle using multiple triangles, there will be an illusion of depth or angle. Therefore, we use multiple triangles to achieve this effect. This method used for drawing shapes using multiple closed triangles is called tessellation. Tessellation is a core concept in computer graphics and is used everywhere from simple 2D graphics to complex 3D environments.
Creating a More Complex Triangle
Now that we know how to make a simple triangle, let’s explore how to make it more complex. We can achieve a more complex triangle by combining multiple triangles to create a mesh. Here is an example of how that can be done:
Using the same set of vertices, we create two triangles that will have a shared edge. To do this, we define our vertices as a fan of triangles where the first vertex is the center of the fan and the remaining vertices are the edges of the triangles as shown in the image above.
Creating a Tessellation of the Triangle Using a Loop
Now that we know how to create a complex triangle, let’s look at how we can make a tessellation using a loop:
Using nested loops, we can create a tessellated triangle. Here is what our code may look like:
---------------------
### Q. What is a vertex?
A vertex is a point or coordinate in space.
### Q. How do you draw a triangle in c++?
To draw a triangle in c++, you need to define your vertices, generate a VAO and VBO, buffer your data, specify vertex attribute pointers, and draw your triangle in a loop.
### Q. Why do we use tessellation?
We use tessellation to create complex shapes using multiple 2D triangles. Tessellation is a fundamental concept in computer graphics and is used for everything from simple 2D graphics to complex 3D environments.
### Q. What is the relationship between the triangle and the tessellation?
A mesh is needed to obtain a more detailed object. It is created by drawing multiple triangles to form a patch of an object. While, the tessellation is mainly used to create meshes using multiple open or closed polygons (most likely triangles).
Conclusion of How to Draw a Triangle in C++
-------------------------------------------
Drawing a triangle is a simple yet fundamental concept in graphics programming. Knowing how to draw a triangle and a more complex tessellated triangle is key to understanding the basics of graphics programming. We hope this article has provided you with the knowledge you need to get started with c++ graphics programming.
Gallery
-------
### Patterns In C++ | Learn Various Patterns In C++ Program
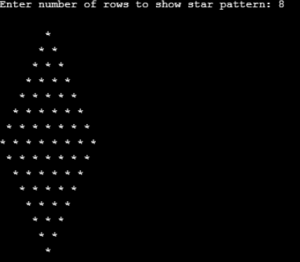
<sub><sup>Photo Credit by: bing.com / educba</sup></sub>
### C++ OpenGL #12: Draw Triangle Fan ~ Take A New Steps In Programming World
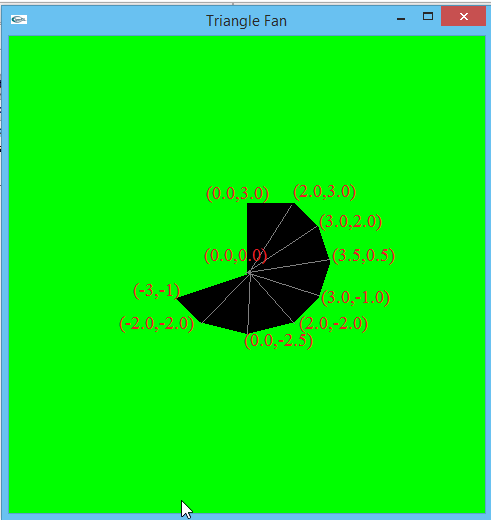
<sub><sup>Photo Credit by: bing.com / triangle fan opengl draw</sup></sub>
### Program To Make Triangle Using Loop In C++ - YouTube

<sub><sup>Photo Credit by: bing.com / triangle loop rgb lights led strip interior music car atmosphere 4x neon usb lamp decorative using program</sup></sub>
### How To Make An Increasing Star Pattern In C++ - Quora

<sub><sup>Photo Credit by: bing.com / </sup></sub>
### C++ Shapes Code ~ C++ Programming Tutorial For Beginners
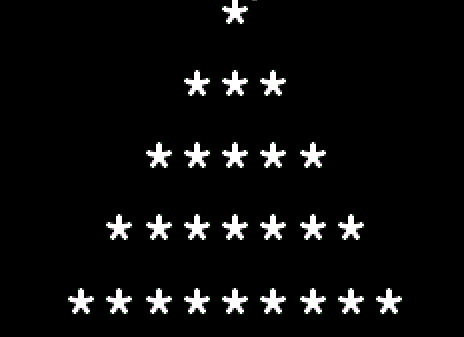
<sub><sup>Photo Credit by: bing.com / code display triangle loop isosceles nested using filled asterisk draw shapes program output write character programming fahad cprogramming shape which</sup></sub>